Integrations
Online Generator
The easiest way to try out JSPM is to generate an import map using the online generator at https://generator.jspm.io.
In the top-left corner enter an npm package name to add to the import map (lit
in this example):
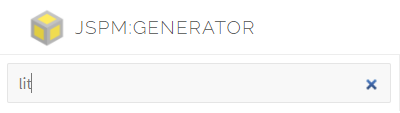
Press Return
to add the package to the map. Then add any other dependency entries. For example to add the ./html.js
subpath export of lit, add lit/html.js
:
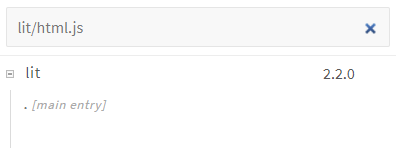
Versions are supported in package names before the subpath and items can be removed or changed from the controls provided.
The final import map is shown on the right, and can be retrieved as an HTML page template or as direct JSON.
In this example we get:
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>Untitled</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
</head>
<body>
<!--
JSPM Generator Import Map
Edit URL: https://generator.jspm.io/#U2VhYGBiDs0rySzJSU1hyMkscTDWM9QzQbD0M0pyc/SyigHdBe16KgA
-->
<script type="importmap">
{
"imports": {
"lit": "https://ga.jspm.io/npm:lit@3.1.4/index.js",
"lit/html.js": "https://ga.jspm.io/npm:lit@3.1.4/html.js"
},
"scopes": {
"https://ga.jspm.io/": {
"@lit/reactive-element": "https://ga.jspm.io/npm:@lit/reactive-element@2.0.4/development/reactive-element.js",
"lit-element/lit-element.js": "https://ga.jspm.io/npm:lit-element@4.0.6/development/lit-element.js",
"lit-html": "https://ga.jspm.io/npm:lit-html@3.1.4/development/lit-html.js",
"lit-html/is-server.js": "https://ga.jspm.io/npm:lit-html@3.1.4/development/is-server.js"
}
}
}
</script>
<!-- ES Module Shims import maps polyfills -->
<script async src="https://ga.jspm.io/npm:es-module-shims@1.10.0/dist/es-module-shims.js" crossorigin="anonymous"></script>
<script type="module">
import * as lit from "lit";
import * as litHtml from "lit/html.js";
// Write main module code here, or as a separate file with a "src" attribute on the module script.
console.log(lit, litHtml);
</script>
</body>
</html>
Saving the HTML template locally and serving over a local server provides a full native modules workflow for working with remote npm packages without needing any separate build steps.
The included ES Module Shims polyfill ensures the import maps still work in browsers without import maps support.
The online generator also provides full support for integrity, preloading and custom providers.
VSCode Extension
For an easy fully local workflow try the JSPM Generator VSCode Extension, which is supported as a Web Extension.
This provides a workflow for writing native HTML imports directly, then post-processing the HTML file to insert the generated import map and polyfill.
With the extension installed, create a new VSCode project, with an app.html
file:
<!doctype html>
<head>
<script type="module" src="./lib/app.js"></script>
</head>
<body>
</body>
Then create a new JS file lib/app.js
:
import lit from 'lit';
console.log(lit);
With the editor focus on app.html
open the VSCode Command Palette (Ctrl + Shift + P
) and select the JSPM: Generate Import Map
command

The first question will be whether to inject preloads and integrity for the generation process, in this case select No
:

This preference can also be saved and changed any time from the VSCode JSPM Settings.
The final question is what environments to generate the import map for. The default generation is with the browser
, development
and module
exports conditions, in this case we select a production
instead of a development
import map:
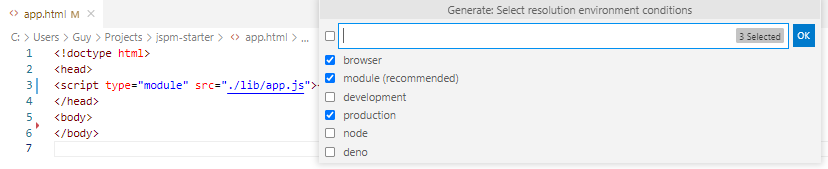
Press return again and the generator will run the complete generation API from within VSCode, modifying the HTML to include the injected import map on completion.
The app.html
file will then be updated by the extension with the ES Module Shims polyfill and import map tags:
<!doctype html>
<head>
<!-- Generated by @jspm/generator VSCode Extension - https://github.com/jspm/jspm-vscode -->
<script async src="https://ga.jspm.io/npm:es-module-shims@1.4.7/dist/es-module-shims.js" crossorigin="anonymous"></script>
<script type="importmap">
{
"scopes": {
"./": {
"lit": "https://ga.jspm.io/npm:lit@2.2.0/index.js"
},
"https://ga.jspm.io/": {
"@lit/reactive-element": "https://ga.jspm.io/npm:@lit/reactive-element@1.3.0/reactive-element.js",
"lit-element/lit-element.js": "https://ga.jspm.io/npm:lit-element@3.2.0/lit-element.js",
"lit-html": "https://ga.jspm.io/npm:lit-html@2.2.0/lit-html.js"
}
}
}
</script>
<script type="module" src="./lib/app.js"></script>
</head>
<body>
</body>
Rewriting will carefully respect existing HTML and existing import mappings. The operation is also largely idempotent unless there are resolution differences.
Version ranges will be consulted from any local package.json
file. The full Node.js resolver rules are supported so it's even possible to use own-name resolution and package imports resolution while respecting custom local import map mappings.
After processing, app.html
should then be a fully executable HTML application. For production it is usually advisable to use the preload option which will inject integrity as well for static guarantees.
Vite Plugin
Vite is architected primarily to modern ES modules workflows, making JSPM and Vite a great combination.
With the JSPM Vite plugin, dependencies are retrieved from JSPM while the project is bundled using Vite.
npm install vite-plugin-jspm --save-dev
vite
and vite build
can be used for dev server and production builds respectively. The plugin takes all the options that are supported with @jspm/generator.
vite.config.mjs
import { defineConfig } from 'vite';
import jspmPlugin from 'vite-plugin-jspm';
export default defineConfig({
plugins: [jspmPlugin()],
});
The plugin will automatically inject ES Module Shims to polyfill import maps.
An additional option that you can use is downloadDeps
, to download and build all the dependencies from the JSPM CDN at build time.
When using the downloadDeps
option, there are a number of factors that should be considered:
- Using external CDN dependencies will likely provide the best latency by utilizing the shared globally distributed CDN network - since the JSPM CDN cache is shared at the edge with other JSPM users this leads to shared latency optimization on edges.
- Using import maps in production results in there being no need to cache bust the entire build. When there is a small change in project, unchanged dependencies remain cached.
- Very minimal footprint of the app as the dependencies are handled by the CDN.
- Performance may be faster with or without
downloadDeps
depending on the exact loading profile and caching requirements. - Having all sources collected together via
downloadDeps
can be useful for a fully self-contained distribution.
Node.js Loader
Status: Experimental
A loader for Node.js to HTTPs imports and import maps with JSPM.
See https://github.com/jspm/node-importmap-http-loader.
Import Map Rails
Import Map Rails provides Ruby on Rails dependency management with import maps and JSPM.
Symfony AssetMapper
Symfony AssetMapper provides dependency management with import maps and JSPM for the Symfony framework in PHP.